While, Do-While and Do-Until loops
Table of Contents
I. Introduction
Let's take a look at How While, Do..Until and Do..While loops work in PowerShell, as they play an important role in your scripts! They're very different from the For and ForEach loops we've already studied.
Although the syntax of these three loops is very similar, if not identical, their logic is different. And that's great fun, because it opens up a whole new world of scripting possibilities! Let's take a look at how these loops work.
This chapter is available in video format:
II. The While loop
A. Logic and syntax
Unlike the "For" loop, which executes a specific number of repetitions, and the "Foreach" loop, which runs through each element of a collection, the "While" loop continues to execute as long as a certain condition is true.
On a line, it is written as follows:
While (condition) { instructions }
While (la condition est vrai){ exécute ce code }
When it comes to logic, it's important to understand that the "While" loop first checks the condition. If the condition is true, it executes the instructions between the braces. It continues to check the condition and execute instructions as long as the condition remains true, so the number of iterations can be variable. This means that if the condition is false from the outset, the instructions are never executed and the script continues to run.
B. Example 1
Let's start with a very simple example, but one that's essential if we're to understand how a "While" loop works in practice. At the start, the variable "$i", which we could have called otherwise, is equal to 0. We're going to display the value of "$i" in the console as long as its value is less than or equal to 20. The condition will therefore be as follows:
$i -le 20
If we integrate this into a "While" loop, we obtain :
# Initialize variable $i to 0
$i = 0
# As long as $i is less than or equal to 20, we display the contents of $i in the console
While ($i -le 20) {
# Display the value of $i in the console
Write-Output $i
# Increment the value of $i by 1
$i++
}
Write-Output "We're out of the While loop!"
At runtime, the condition"$i -le 20" is true, so the loop will execute. After each iteration of the loop, the value of"$i" is incremented by 1. When"$i" reaches 21, the condition"$i -le 20" will become false, so the loop will stop!
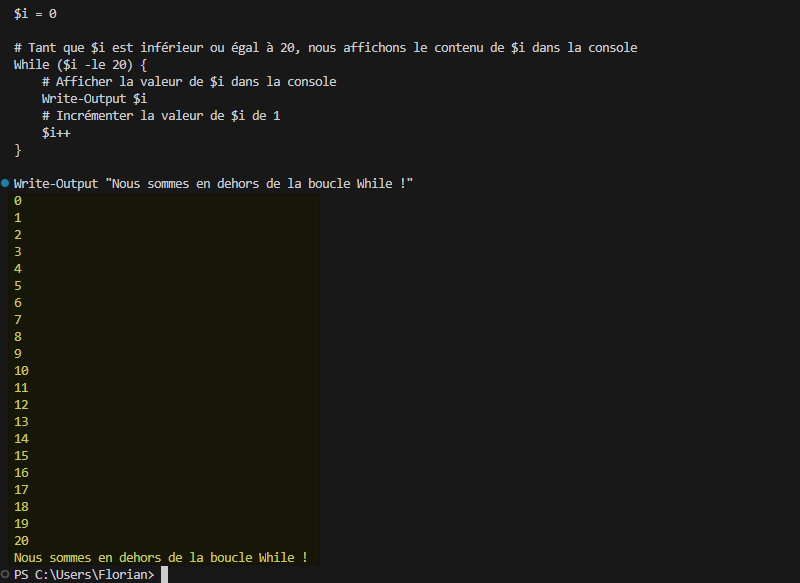
If you replace"$i = 0" with"$i = 21", then you'll see that the code contained in the While loop will never be executed, since the condition is evaluated before the code is executed.
B. Example #2
We'll use the "While" loop in a second example. This time, our script will wait, thanks to the While loop, until the process of a specific application, for example "notepad.exe" (Notepad), has finished before continuing to execute the rest of the script. So, each time, if the notepad.exe process is still running, we'll trigger a 3-second pause, then re-evaluate the condition, and so on.
# Recover the "notepad" process (hide the error that will be returned if it is not running)
$Processus = Get-Process "notepad" -ErrorAction SilentlyContinue
# As long as the "notepad" process is running, we wait 1 second.
While ($processus) {
# Display a message in the console - Process still running
Write-Output "The notepad application is still running."
# Wait 3 seconds before checking again
Start-Sleep -Seconds 3
# Check whether the "notepad" process is running
# Mandatory line, otherwise the value of the $Process variable will never change!
$Processus = Get-Process "notepad" -ErrorAction SilentlyContinue
}
# End of loop - Validation message
Write-Output "The notepad application is no longer running. The script can continue to run."
The result could be something like this:
# Notepad open on computer
The notepad application is still running.
The notepad application is still running.
The notepad application is still running.
# Closing Notepad on the computer
The notepad application is no longer running. The script can continue to run.
To learn more about this topic, read this article:
III. The Do While loop
A. Logic and syntax
The "Do-While" loop is very similar to the "While" loop, except that its syntax is slightly different thanks to the introduction of the "Do". This is not neutral, as it means that the condition is evaluated after the code in the loop has been executed. In other words, the code will necessarily be executed once, since the condition is evaluated afterwards, whereas with the "While" loop, the condition is evaluated beforehand.
The "Do-While" loop first executes the instructions in the loop, then checks the condition. If the condition is true, it executes the instructions again. We can even say that it continues to execute the instructions and check the condition as long as the condition is true. If the condition is false, the loop is stopped as with "While" alone.
The "Do-While" loop follows the following logic:
Do{code} While(condition is true)
In other words:
Play{this code} As long as (condition is true)
In the "Do While" loop, we say "I'll play the code as long as the condition is true", whereas in the "While" loop we say "As long as the condition is true, I'll play the code".
B. Example 1
We'll use the same example as for the "While" loop, but write it as a "Do-While" loop. At the start, the "$i" variable, which we could have called something else, is equal to 0. We're going to display the value of "$i" in the console as long as its value is less than or equal to 20.
# Initialiser la variable $i à 0
$i = 0
# Exécuter les instructions, puis vérifier si $i est inférieur ou égal à 20
do {
# Afficher la valeur de $i dans la console
Write-Output $i
# Incrémenter la valeur de $i de 1
$i++
} while ($i -le 20)
Write-Output "Nous sommes en dehors de la boucle Do-While !"
The logic is slightly different, but we get the same result:
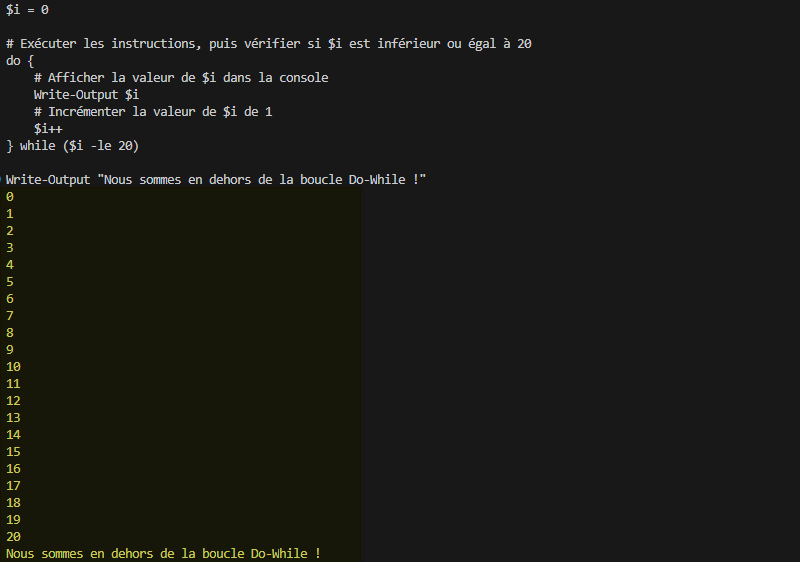
Finally, to choose between "While" and "Do-While", ask yourself the following question: do I want the code in the loop to be executed at least once?
B. Example #2
Here's a second example. Here, we'll initialize the "$i" variable to 0, and as long as the Notepad application is running, we'll indicate the value of "$i" in the console. At each iteration, "$i" is incremented by 1.
# Initialize variable $i to 0 $i = 0 # Execute instructions as long as the notepad process is running Do{ $i++ Write-Output $i }While(Get-Process -Name "notepad" -ErrorAction SilentlyContinue) Write-Output "The Notepad application is closed, we can continue!"
We can see in the console that we exit the loop as soon as I close the Notepad application on my machine:
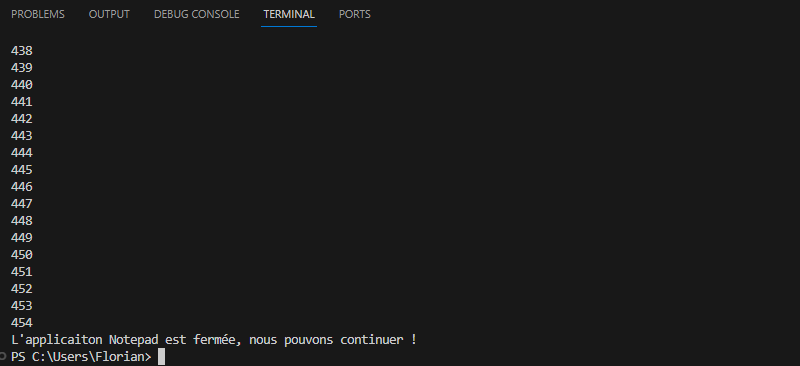
IV. The Do Until loop
A. Logic and syntax
The "Do-Until" loop is a third important control structure in PowerShell. Although it is similar to the "Do-While" loop, there is one major difference: instead of repeating instructions as long as the condition is true (as in "Do-While"), the "Do-Until" loop repeats instructions until the condition becomes true.
The "Do-Until" loop in PowerShell works according to the following logic:
Do{code} Until(condition is true)
In other words:
Play {this code} until (condition is true)
This can be particularly useful for checking a state in the "Do", and as soon as the expected state arrives, you exit the loop. There are many uses for this type of loop, for example as a timer in a script to wait for a state to switch to the expected state before continuing. To obtain the same behavior as with a "Do-While" loop, you need to reverse the logic of the condition.
B. Example 1
We'll use the same first example as for the "While" and "Do-While" loops, but adapt it as a "Do-Until" loop. At the start, variable "$i", which we could have called something else, is equal to 0. We're now going to display the value of "$i" in the console as long as its value is less than or equal to 20.
It's relatively quick and easy, since all you have to do is replace the "While" keyword with "Until", then invert the condition using a different comparison operator. Here, we'll repeat the loop until(until) "$i" is greater than 20, since we want to have values from 0 to 20.
# Initialiser la variable $i à 0
$i = 0
# Exécuter les instructions, puis vérifier si $i est inférieur ou égal à 20
do {
# Afficher la valeur de $i dans la console
Write-Output $i
# Incrémenter la valeur de $i de 1
$i++
} until ($i -gt 20)
Write-Output "Nous sommes en dehors de la boucle Do-Until !"
It's the same result and, more importantly, it's the result we've been waiting for.
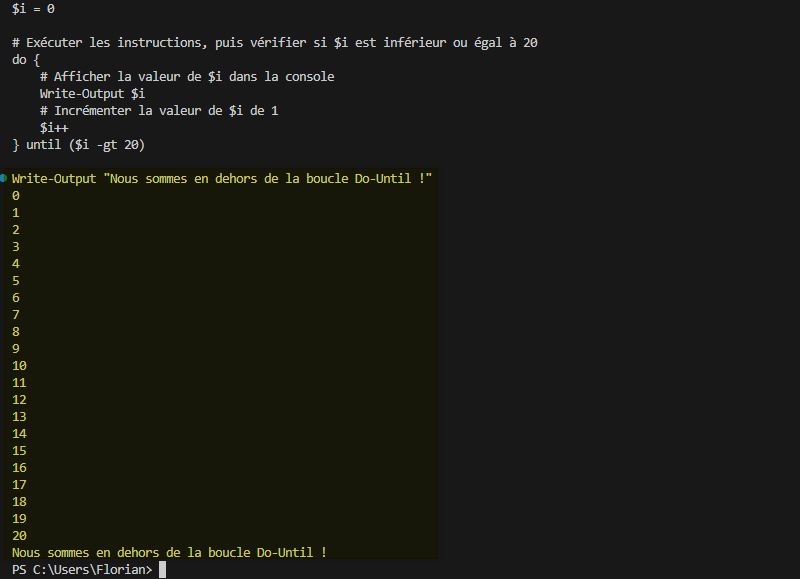
C. Example #2
In this second example, we'll see that we can add several conditions to the "Do-Until", "Do-While" and "While" loops. Let's say we also have a variable "$j" which increments by 1 on each loop turn... Like variable "$i". However, we're going to initialize this variable to 5, whereas "$i" is initialized to 0. We want to exit the loop as soon as "$i" or "$j" reaches 10 :
# Initialize variable $i to 0 $i = 0 # Initialize variable $j to 5 $j = 5 # Execute instructions, then check if $i is less than or equal to 20 do { # Display the values of $i and $j Write-Output "i = $i - j = $j" # Increment the value of $i and $j by 1 $i++ $j++ } until (($i -gt 10) -or ($j -gt 10)) Write-Output "We're out of the Do-Until loop!"
You'll notice that we enclose each condition in parentheses, just as we do in a conditional structure with If. In addition, we use the "-or" operator, but we could also use another operator such as "-and", depending on what we want to do.
Here is the result obtained:
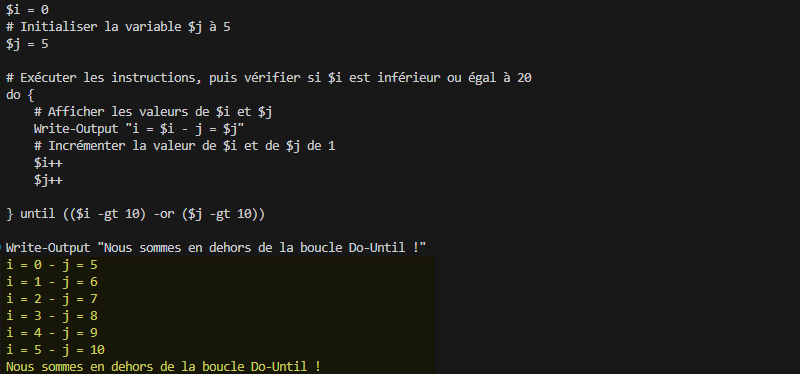
We exit the loop as soon as "$j" exceeds 10, even if "$i" hasn't yet reached this number.
V. Conclusion
The "While", "Do-While" and "Do-Until" loops are also very practical and perfectly suited to certain scenarios, as we have seen.
Another very interesting use case is when we ask an operator to enter information, for example, a password: we can check the length and complexity of the string, and if it doesn't meet our requirements, we can ask for a new value to be entered until it does. You see, in writing this requirement, I used the term "Until" so we could translate it with a "Do-Until" loop, or "Do-While", for that matter.