How to Create a Folder or Multiple Folders with PowerShell?
Table of Contents
I. Introduction
In this chapter, we will learn how to create a folder with PowerShell using the “New-Item” command! This is the native PowerShell command to help you forget the MS-DOS “mkdir” command!
II. Create a Folder with PowerShell
The “New-Item” command, which is also used to create other elements such as files, will be our ally to create one or more folders. Let's start with the basics: creating a folder, specifying its destination path. Nothing more, nothing less.
Here's the command to execute to create the “PowerShell” folder under “C:\TEMP”:
New-Item -Path “C:\TEMP” -Name “PowerShell” -ItemType Directory
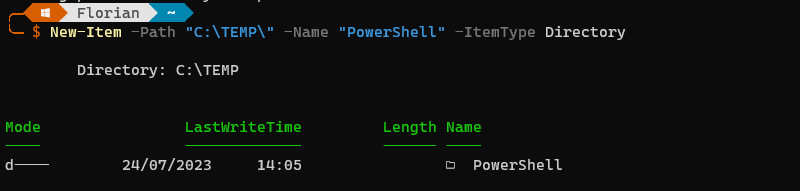
Some explanations are in order:
- -Path: destination path, i.e., the location where the folder should be created
- -Name: the name of the folder
- -ItemType: the type of item, it's very important to specify
Directory
because otherwise, a file without an extension will be created!
With this syntax, we clearly distinguish the path on one side and the folder name on the other.
However, we can make it shorter by incorporating the folder name directly into the path. Personally, I recommend using the model above.
New-Item -Path “C:TEMPPowerShell” -ItemType Directory
If you want to create a folder in the current location, it's not necessary to enter the path of the current directory. In fact, you can simply use this syntax:
New-Item -Name “PowerShell” -ItemType Directory
If we don't specify a value for the “-Path” parameter, the current directory (relative to the PowerShell prompt) will be used.
Other possible syntaxes:
New-Item -Path . -Name “PowerShell” -ItemType Directory New-Item -Path “.PowerShell” -ItemType Directory
There you have it, you are now capable of creating a folder with PowerShell!
III. Create Multiple Folders with PowerShell
Sometimes, we need to create multiple folders, or even a folder tree structure with a parent folder and subfolders. We could chain several New-Item commands (one per folder) to achieve our goal, but we'll see that we can do it more simply to optimize our code!
A. Create Multiple Folders in the Same Location with PowerShell
Rather than typing the New-Item command multiple times, we can specify all the paths and names of the directories to create at the “-Path” parameter level. To create the directories “PowerShell1”, “PowerShell2”, and “PowerShell3” at the root of “C:TEMP”, it looks like this:
New-Item -Path “C:TEMPPowerShell1”,“C:TEMPPowerShell2”,“C:TEMPPowerShell3” -Itemtype Directory
Of course, we could create three folders in different locations...
Let's take another example: creating a list of folders based on data from a CSV file. Here, a CSV file with a single header named “Username”. The objective is simple: create a folder per user, using the value of “Username” as the folder name, within “C:TEMPPowerShell”.
Here's the content of the “Username.csv” file:
Username
Florian
Théo
Adrien
Bobby
We start by importing the CSV file:
$Username = Import-CSV -Path “C:TEMPUsername.csv”
Then, we loop through the content of the CSV file with “ForEach” to create a directory for each username. Each line of the CSV file will be processed by reading the “$Username” variable.
$Username | foreach { New-Item -Path “C:TEMPPowerShell” -Name $_.UserName -ItemType Directory }
Which gives the expected result:
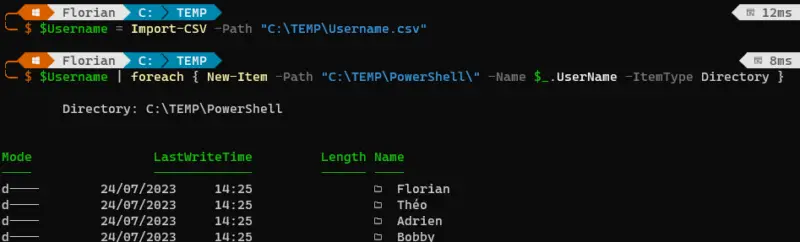
We could make the syntax shorter by avoiding using an intermediate variable:
Import-CSV -Path “C:TEMPUsername.csv” | foreach { New-Item -Path “C:TEMPPowerShell” -Name $_.UserName -ItemType Directory }
You are able to create multiple folders with PowerShell!
B. Create a Folder Structure with PowerShell
Sometimes, we need to create a root folder and subfolders: how to do this with PowerShell? This is what we will cover in this part of the chapter.
First, you should know that by default the New-Item command allows you to create the complete path. This means that if we want to create the folder “Scripts” in “C:TEMPPowerShell”, but the “PowerShell” directory doesn't exist, it will be created automatically! Thus, we will create a directory structure without intending to.
New-Item -Path “C:TEMPPowerShellScripts” -ItemType Directory
The example below will create the folders “Documents”, “Downloads”, and “Private” in the root folder of each user (from the list of names in the CSV file).
$Dossiers = ( “Documents”, “Downloads”, “Private” ) Import-CSV -Path “C:TEMPUsername.csv” | foreach { $Chemin = “C:TEMPPowerShell$($_.UserName)” $Dossiers | Foreach { New-Item -Path $Chemin -Name $_ -ItemType Directory } }
We can see that the three folders are indeed created each time:
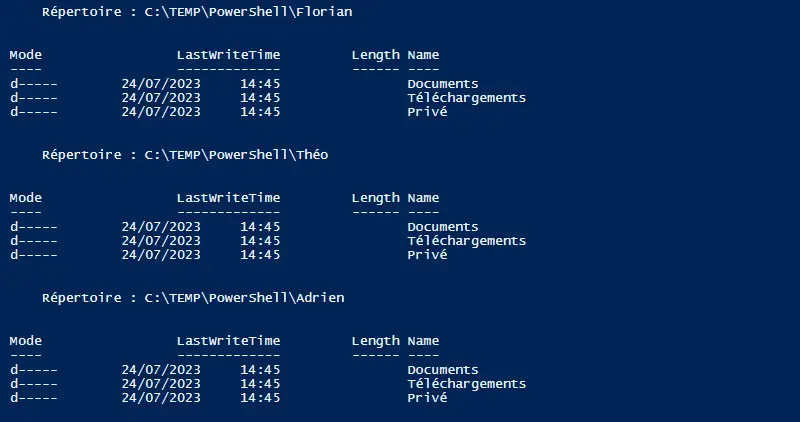
Let's finish with a tip based on using the asterisk “*”! Let's assume we have a list of directories created after reading the CSV file (previous example):
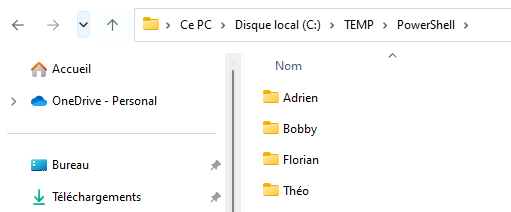
If we want to create a subfolder “Documents” in all folders contained at the root of “C:TEMPPowerShell”, how do we do it? We could get the list of folders with “Get-ChildItem” and execute the New-Item command each time to create the missing folder... But, we can do it much more simply:
New-Item -Path “C:TEMPPowerShell*” -Name “Documents” -ItemType Directory
Which gives the expected result:
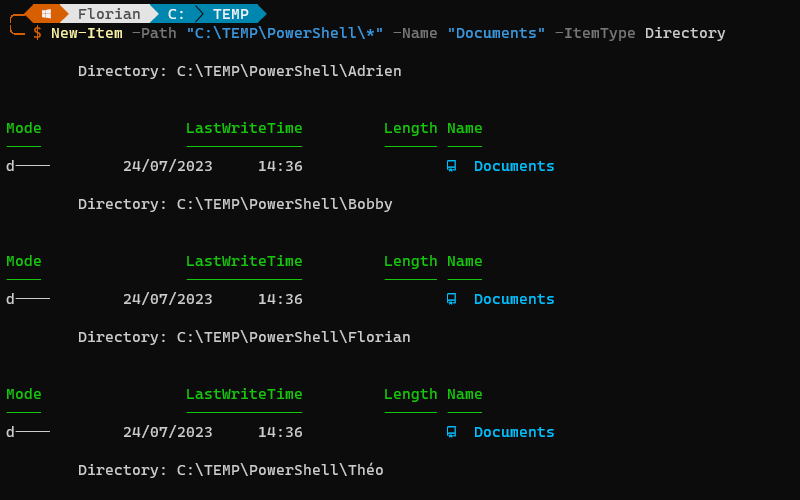
Placing the asterisk at this location will create the “Documents” folder in all subdirectories! Good to know, but use with caution...
IV. Conclusion
You are now able to create one or more folders with PowerShell, whether from a static list or even from the contents of a CSV file! All that's left is for you to take advantage of the New-Item
command!
