How errors work in PowerShell
Table of Contents
I. Introduction
When you write your PowerShell scripts, you're bound to encounter errors. Even perfectly written, best-practice code is liable to generate errors: an unexpected value for a cmdlet parameter, a poorly written command, a problem with computer rights , an unauthorized operation, etc. There are many situations in which an error can occur... And we need to be able not only to interpret these errors, but also to handle them.
In this first chapter, we're going to take a look at how errors work in PowerShell, as this will be essential for what follows, since in the next chapter we'll be looking at how to handle errors with "Try-Catch and Finally".
In addition to the automatic "$?" variable, which indicates whether or not the last command was executed correctly (a Boolean is returned), PowerShell provides us with the "$Error" variable, as well as the "Get-Error" cmdlet.
II. Fatal and non-fatal errors
In PowerShell, there are two main types of error: fatal errors and non-fatal errors, also known as blocking and non-blocking errors. In English, there are two associated terms: terminating error and non-terminating error.
Fatal errors are serious errors that stop script execution immediately. They can occur in a variety of situations, and a cmdlet will not always return a fatal error. This error can occur if a problem is encountered that blocks further execution.
Conversely, as you may have guessed, non-fatal errors do not stop script execution. They simply indicate that an error has occurred, but the script continues to run. Handling these two types of error is essential in your scripts.
III. The $Error variable
When working with PowerShell, it's important to understand how to use and interact with the "$Error" variable. This PowerShell-managed variable plays a crucial role, as we'll be using it to retrieve the latest errors, which will be very useful in the debugging phase. It contains both fatal and non-fatal errors.
First of all, you should know that the "$Error" variable is an automatic variable that contains an array of the most recent errors that occurred during the execution of a script or command. The most recent error is always at index 0 of the array. Each time an error, also known as an exception , is generated, it is added to the "$Error" array.
Its contents are ephemeral, as it is cleared at the end of each PowerShell session. In other words, if you close the PowerShell console and reopen it,"$Error" will be empty.
In practice, the "$Error" variable can be used to obtain detailed information on errors. You can display all errors by simply typing "$Error" in the console. You can also access a specific error by specifying its index number.
Execute the two commands below to generate two errors:
Get-Contenu -Path "C:tempfichier.txt"
Get-Content -Path "C:tempfichier.txt"
In the first case, the cmdlet name is incorrect, while in the second, the file cannot be found.
We can consult the"$Error" variable to display these two errors:
$Error
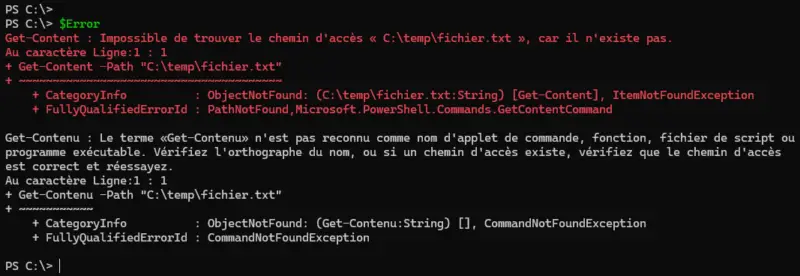
Then, if we only want to retrieve the most recent error (or one specific to its index) :
$Error[0]
Rather than retrieving the entire error message, we can retrieve specific information.
- Retrieve only the text corresponding to the error:
$Error[0].Exception.Message
Here, it will be:"Impossible to find the path "C:tempfichier.txt", because it doesn't exist.".
- Retrieve the line number from the script :
$Error[0].InvocationInfo.ScriptLineNumber
Here, it will be "1" because it's a command executed in the console, not a script.
- Retrieve the type of error generated :
$Error[0].Exception.GetType().FullName
Here, the value returned is:"System.Management.Automation.ItemNotFoundException".
Each time, depending on the information we want to retrieve, we'll read a different property.
IV. The Get-Error cmdlet
Since PowerShell 7, we've been able to use the"Get-Error" cmdlet to obtain information on the latest errors encountered in the current session. The"Get-Error" cmdlet is used to obtain a "PSExtendedError" object, which is fed from the information contained in "$Error".
Get-Error
The result returned by "Get-Error" is formatted, compared to the direct reading of "$Error".
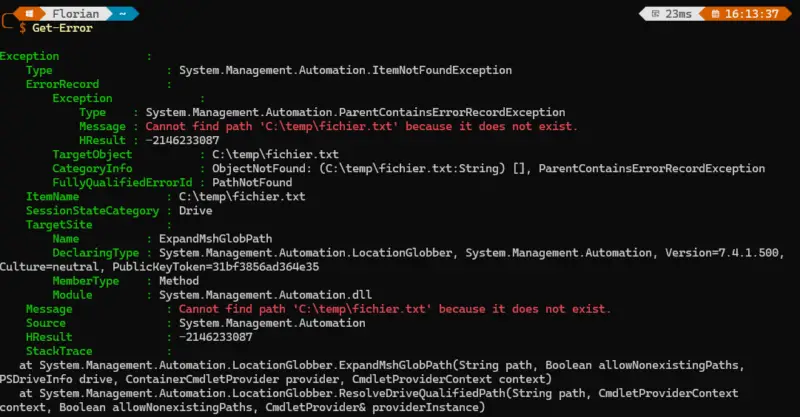
A useful feature of "Get-Error" is the"-Newest" parameter, which allows you to obtain the X most recent errors. For example, to obtain the three most recent errors, we simply need to execute this command:
Get-Error -Newest 3
V. The $ErrorActionPreference variable
"$ErrorActionPreference" is a preference variable built into PowerShell which determines how it should handle non-blocking errors. Several values are valid, and the default value is"Continue".
The following values are supported: SilentlyContinue, Stop, Continue, Inquire, Ignore, Suspend and Break. Note that the"Break" value is not supported by Windows PowerShell, and that the"Ignore" and"Suspend" values are supported only by the"-ErrorAction" parameter, which we'll look at next.
Value | Description |
---|---|
SilentlyContinue | Errors are removed and the script continues to run. |
Stop | Script execution is stopped as soon as an error occurs. |
Continue | Errors are displayed in the console, but the script continues to run. Default value. |
Inquire | PowerShell asks the user if he wants to continue each time an error occurs. |
Ignore | The error returned by the command is ignored and not even added to the $Error variable. |
Suspend | For PowerShell workflows only, to suspend it on error to allow debugging. |
Break | When an error occurs, PowerShell activates the debugger on the instruction currently being executed. |
To define another value, assign a new value to the variable :
$ErrorActionPreference = "stop"
The value of the "$ErrorActionPreference" variable can be modified in the PowerShell profile to be persistent, or directly in a PowerShell console to apply only while the console is open, or at the start of a script. However, it is recommended to use the "-ErrorAction" parameter.
VI. The ErrorAction parameter
The"-ErrorAction" parameter is a common parameter you can use with any cmdlet in PowerShell, including a function you've developed if it benefits from the CmdletBinding() parameter.
This parameter lets you specify the behavior of the executed command if a non-blocking error occurs during execution. The values you can assign to "-ErrorAction" are the same as those for the "$ErrorActionPreference" variable, namely:"SilentlyContinue", Stop, Continue, Inquire, Ignore, Suspend and Break.
For example, if you use"Stop" as the value, a fatal error will be generated and script execution will be stopped (unless a "Try-Catch" instruction block is used to catch the error). If you use"SilentlyContinue", the cmdlet will ignore the error and the script will continue to run. This parameter is very useful for handling errors directly at cmdlet level, individually and independently, within a PowerShell script.
Here's how to use this setting:
Get-Content -Path "C:tempfichier.txt" -ErrorAction Stop
VII. Conclusion
Now that you know more about how errors work in PowerShell, let's learn how to handle them using Try, Catch and Finally.