Get-Date : manipulating date and time in PowerShell
Table of Contents
I. Introduction
PowerShell features a native cmdlet for retrieving the date and time on a machine: "Get-Date". Behind this easy-to-remember command name lies an indispensable tool for our PowerShell scripts, which you'll find yourself using in many situations. You can use it to write the date and time to a log file created by your script, to compare the current date with the date of an object (date of last access to a file, user creation date, etc.), or to perform calculations on dates.
II. Formatting date and time with PowerShell
First, we'll use the "Get-Date" cmdlet to format the date and time in different ways. Let's start by running this command without any parameters to get an idea of the default format.
Get-Date
The default output format is: Wednesday, April 3, 2024 17:29:43
With the"-Format" parameter, we can customize the format of this command's output by using a set of characters ("m", "d", "y", "h", "s", "f") in upper or lower case (case is important and affects the returned result).
Here are a few examples:
- Get the date in the form "YearMonthDay":
Get-Date -Format"yyyyMMdd" 20240403
In fact,"yyyy" gives the year, while "MM" gives the month in numeric format and "dd" the day in numeric format.
- Get the date in "Day/Month/Year" format:
Get-Date -Format"dd/MM/yyyy" 03/04/2024
- Get the current time, with minutes, without seconds:
Get-Date -Format"HH:mm" 17:32
Don't confuse"mm" and"MM", as the former is used to retrieve minutes, while the latter is used to retrieve the month in digital format.
- Get the month spelled out :
Get-Date -Format"MMMM" april
- Get date and time separated by an underscore in the form "YearMonthDay_TimeMinute" :
Get-Date -Format"yyyyMMdd_HHmm" 20240403_1734
- Get only the time with seconds:
Get-Date -DisplayHint Time 17:34:28
- Get date only (here, in its default format) :
Get-Date -DisplayHint Date Wednesday, April 3, 2024
You can create a file, a folder or any other element that contains the date in its name. The following example will create a file in "C:TEMP" with the following name: Log_Date_Heure.txt. For example: Log_20240404_0812.txt. We could also store this information in a variable and call up the variable.
New-Item -Path "C:TEMPLog_$(Get-Date -Format 'yyyyMMdd_HHmm').txt" -ItemType File
With these few examples, you should be able to find a format that suits your needs.
III. Setting a static date
To statically retrieve a date and store it in a variable to inherit the correct type ("DateTime"), we can directly exploit the parameters of the "Get-Date" command, like this:
$StaticDateTime = (Get-Date -Year 2024 -Month 04 -Day 03 -Hour 00 -Minute 00 -Second 00)
The alternative is to use this syntax :
[datetime]$DateStatique = "04/03/2024"
This forces the "DateTime" type, otherwise the text is considered astring. In this case, the value "04/03/2024" gives the date April 3, 2024 (pay attention to the month and day order).
IV. Calculate a date
In a script targeting the Active Directory, for example, we might want to obtain a list of user accounts not used in the last 90 days. To do this, we'll need to obtain a variable whose value will be the date 90 days ago, on a sliding scale since this date will change every day.
To calculate a date, we'll use the "AddDays()" method, which applies directly to "Get-Date" and expects a number as value. If the number is positive, the number of days will be added to the current date; if it is negative, it will be subtracted and the calculated date will be returned.
Subtracting 90 days from today's date gives us :
(Get-Date).AddDays(-90)
For example:
Write-Output "Today's date: $(Get-Date)" Write-Output "Date 90 days ago: $((Get-Date).AddDays(-90))" Write-Output "Date in 90 days: $((Get-Date).AddDays(90))"
Which returns :

Now that your reference date has been calculated, you can easily compare this date with another! A condition in your script with a comparison operator such as "-lt" or "-gt" will be very effective for finding out whether one date is "bigger" or "smaller" than another.
Note: If you need to add or subtract hours, minutes or seconds, you can! Simply use the appropriate method: AddHours(), AddMinutes(), AddSeconds() or AddMonths() for months.
V. Convert date to timestamp
Atimestamp is a numerical value whose purpose is to represent the date and time in a specific format. In some cases, you may need to compare two timestamps. If you have a timestamp on one side and the date/time in another format on the other, this poses a problem.
It is then necessary to convert the "date-time" string into a timestamp value. To achieve this, we'll use the "ToFileTime()" method, accessible directly in PowerShell.
This gives us :
(Get-Date).ToFileTime() 133566335296961495
The value returned is a timestamp. This value is incomprehensible to humans, but not to machines.
VI. Convert string to date
Let's take a previously shared example and see how it's possible to convert a string into a date, i.e. into "DateTime" format, using a method called "ParseExact()", and the .NET "DateTime" class.
$DateStatique = "04/03/2024"
If we look at the type of this variable, we can see that it's assigned the "string" type. This does not correspond to our expectations.
$DateStatique.GetType()

The "ParseExact" method of the "DateTime" class expects three values: the date in text form, the desired output format, and a provider (we'll use $null).
[DateTime]::ParseExact("<Date>","<Format>",$null)
So, to update our "$DateStatic" variable from a "String" type to a "DateTime" type, we'll use the following:
$DateStatique = [DateTime]::ParseExact("$DateStatique","MM/dd/yyyy",$null)
Here, we specify the format "MM/dd/yyyy" because our date is written in the form "Month / Day / Year", but of course we need to adapt this to the format of the string sent as input.
We obtain the expected result:
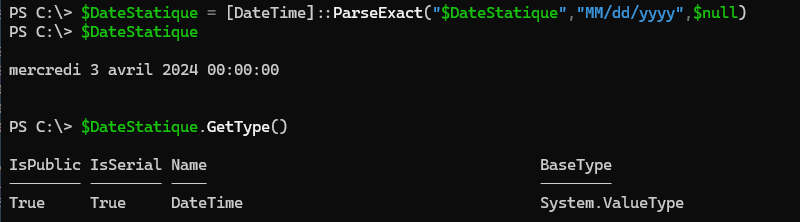
We could also use this syntax :
$DateStatique = [DateTime]::ParseExact("04/03/2024","MM/dd/yyyy",$null)
In addition to date and time, the "Get-Date" command can be used to obtain other calendar-related information directly, for example: how do I know what day of the year it is? PowerShell can answer this question.
(Get-Date).DayOfYear 94
We can obtain other information, such as the number of the current month:
(Get-Date).Month 4
It's also possible to obtain details about the current day: how many seconds have elapsed today, or even milliseconds? This command makes it easy!
(Get-Date).TimeOfDay Days : 0 Hours : 18 Minutes : 0 Seconds : 42 Milliseconds : 264 Ticks : 648422642098 TotalDays : 0,750489169094907 TotalHours : 18,0117400582778 TotalMinutes : 1080,70440349667 TotalSeconds : 64842,2642098 TotalMilliseconds : 64842264,2098
VIII. Conclusion
After reading this chapter, you have a good basis for manipulating date and time in your PowerShell scripts! If you want to go further, you can consult the help for this cmdlet and list properties and methods using "Get-Member".