Discovering PowerShell modules
Table of Contents
I. Introduction
In this chapter, we'll look at the notion of the PowerShell module to answer a number of questions: What is a PowerShell module? Where can I find modules? What are the most popular modules? Or how do you install a module on your machine?
Before getting to the heart of the matter, each PowerShell cmdlet is associated with a PowerShell module. This concept has been integrated into PowerShell 2.0.
II. What is a PowerShell module?
A PowerShell module is a group of cmdlets associated with a Windows operating system component, service or application. Let's take a very evocative example: the PowerShell "Active Directory" module contains all the cmdlets needed to interact with an Active Directory, whether to create users or groups, retrieve the list of computers, etc...
Modules bring flexibility to PowerShell, because even though Windows comes with a set of modules, this allows each system administrator to install the modules they need.
Here are just a few of the modules natively present in Windows :
- PowerShellGet , a collection of cmdlets for managing modules and repositories (sources)
- BitLocker , a collection of cmdlets for managing BitLocker encryption on Windows
- NetAdapter , a collection of cmdlets for managing network interfaces
- DnsClient , a collection of cmdlets for interacting with the Windows DNS client
- Etc...
In fact, you can list all the modules installed and available on your computer with this command:
Get-Module -ListAvailable
In addition, here's how to list all the commands present in a specific module:
Get-Command -Module <Module Name>
Get-Command -Module BitLocker
Here's an example:
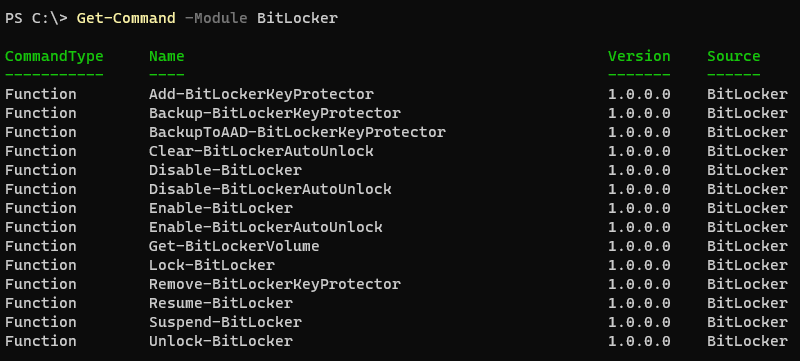
You should be aware that modules are stored locally on your machine and that there is a notion of versioning. This means that modules evolve over time and that the maintainer is likely to publish new versions to correct a bug, add a parameter to a cmdlet, add a new cmdlet, etc...
Where are the modules stored?
To answer this question, we need to read the contents of the following environment variable:
$env:PSModulePath
Here is an example of a value:
C:Usersdemo-DocumentsPowerShellModules;C:Program FilesPowerShellModules;c:program filespowershell7Modules;C:Program FilesWindowsPowerShellModules;C:Windowssystem32WindowsPowerShellv1.0Modules
We can identify several directories, whose names are separated by a semicolon, and which refer to the locations in which you can store modules.
III. PowerShell Gallery, the main repository
The worldwide PowerShell community is very large. On the one hand, there are the PowerShell modules developed and made available by Microsoft, and on the other, the PowerShell modules created by members of the community.
A few years ago, Microsoft had the good idea of launching a site called "PowerShell Gallery". It can be accessed at powershellgallery.com, and its aim is to bring together all the resources available to the community.
As a result, this has become the main repository for finding a PowerShell module and obtaining information about it: name of developer, version history, etc. Even so, some modules are not referenced here and can be accessed by other means: a GitHub repository, for example.
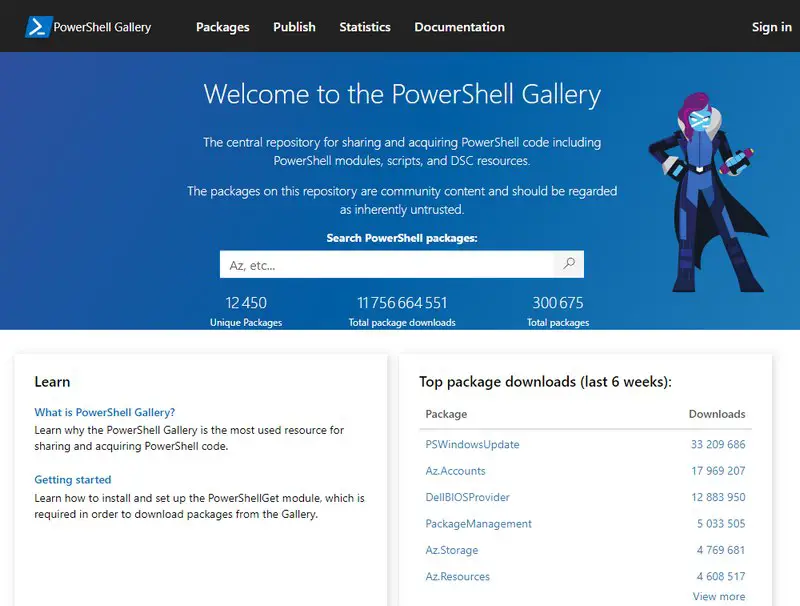
We can find many modules, including PSWindowsUpdate which manages the Windows Update service via PowerShell, and is currently the most popular PowerShell module.
When we click on a module, we arrive on a dedicated page with a wealth of valuable information:
1 - The name of the module or resource, with the current version.
2 - The minimum version of PowerShell you need to use this module.
3 - The module installation command.
4 - Module author. This is a community module. In some cases, Microsoft is specified.
5 - Version history, with release date and number of downloads.
6 - Statistics: number of downloads, date of most recent version.
7 - Some useful links, including "Project Site", which usually links to the GitHub repository associated with the resource.
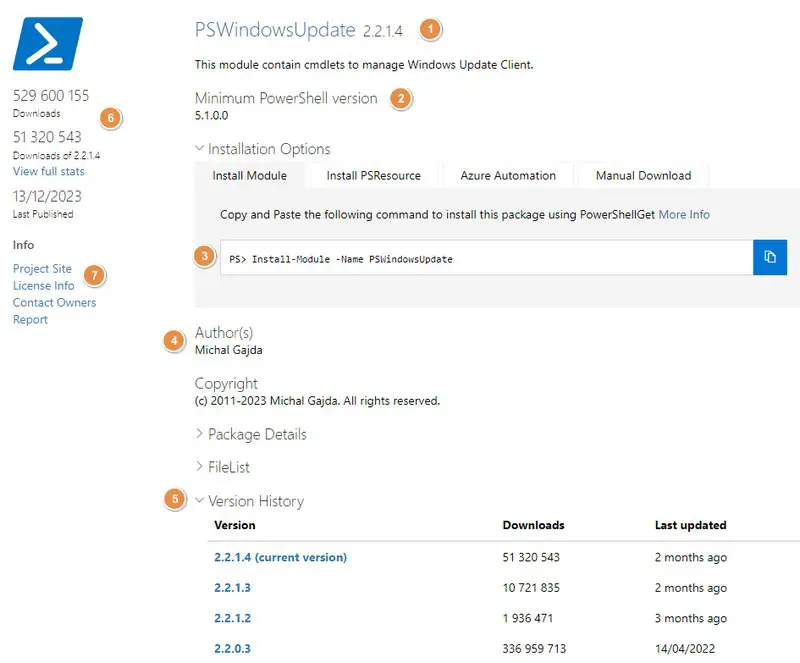
This information should be taken into consideration when assessing the popularity and reliability of a module.
Microsoft uses the PowerShell Gallery to publish certain modules, particularly those that interact with Microsoft 365 services and the Azure Cloud, including: PowerShell Graph, MicrosoftTeams, ExchangeOnlineManagement.
In addition, some PowerShell modules are available as optional features from the Windows or Windows Server operating system. For example, the Active Directory module is integrated into Windows Server, but is not installed by default.
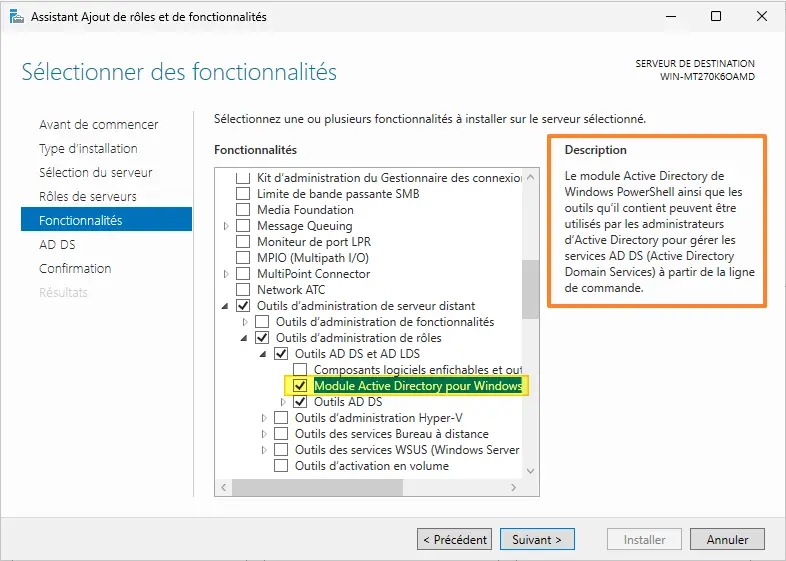
IV. Installing and updating a PowerShell module
In this chapter, we'll look at how to install a PowerShell module from the PowerShell Gallery. We'll also look at how to update a PowerShell module.
A. Installing a PowerShell module
To install a PowerShell module, we can use the "Install-Module" cmdlet to download and install a module from a repository. By default, only one repository is declared on Windows: the PowerShell Gallery, aka PSGallery.
We can check this with the following command:
Get-PSRepository
Here's the result. We can see that this repository is considered "Untrusted". This means you'll have to validate it manually when you want to install a PowerShell module from this source.

We're going to install the "PSWindowsUpdate" module, which interacts with the Windows Update component. The "Install-Module" cmdlet works like this:
Install-Module -Name <Module Name>
Install-Module -Name PSWindowsUpdate
We can see the "Untrusted repository" security alert being displayed, concerning the PSGallery repository. We need to validate this installation by indicating "Y" to validate.

A few seconds later, the PowerShell module will be installed on your machine!
Note : by default, PowerShell will install the latest stable version of a module. You can install a specific version by adding the "-RequiredVersion" parameter followed by the version number. In addition, the "-AllowPrerelease" parameter authorizes installation of a "Preview" version still under development.
To verify this, we can run various commands. Here are a few examples, which will enable you to manipulate and discover additional cmdlets.
- Search for the module by name in the list of installed modules :
Get-Module -Name PSWindowsUpdate -ListAvailable
- List all PowerShell modules installed on the local computer via PowerShellGet :
Get-InstalledModule
- Display the list of commands present in this module :
Get-Command -Module PSWindowsUpdate
- Import PowerShell module (no feedback means successful operation) :
Import-Module -Name PSWindowsUpdate
This last command is used to import a PowerShell module into the active PowerShell session. However, it has lost some of its usefulness since the release of PowerShell 3.0, as installed modules are automatically imported into the session whenever commands present in this module are called. In other words, the import process is transparent to the user.
For your information, you can approve the PSGallery repository with this command (although I recommend that you leave this confirmation step as a precaution):
Set-PSRepository -Name PSGallery -InstallationPolicy Trusted
B. Updating a PowerShell module
To update a PowerShell module, use the"Update-Module" cmdlet. Note that on a local machine, you may have several versions of the same module.
To put the module update into practice, we'll uninstall the "PSWindowsUpdate" module from our machine, then reinstall an older version, then trigger the update to the latest version.
Start by uninstalling the "PSWindowsUpdate" module if you have installed it:
Uninstall-Module -Name PSWindowsUpdate
Next, we will install version 2.2.1.2 of this module:
Install-Module -Name PSWindowsUpdate -RequiredVersion 2.2.1.2
Once this is done, we'll update the :
Update-Module -Name PSWindowsUpdate
There are now two versions of this module on our machine:
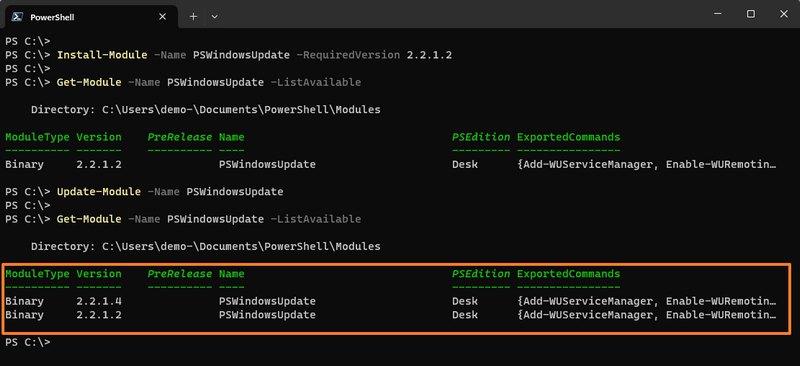
We need to make the effort to uninstall the old versions. To remove old versions of a module and keep only the old one, you can use the 3 lines of code shown below. This will be an opportunity to put into practice a concept we've already studied: the pipeline, and to take our first step in variable manipulation.
$ModuleName = "PSWindowsUpdate"
$ModuleLatestVersion = Get-InstalledModule $ModuleName
Get-InstalledModule $ModuleName -AllVersions | Where-Object {$_.Version -ne $ModuleLatestVersion.Version} | Uninstall-Module
Finally, please note that the command below will update all the modules you have installed on your machine. A pity, however, is that this command does not indicate the current version of the module, or even the version of the module to be installed (and its release date). To go further, you'll need to rely on a more comprehensive update script, such as this one available on GitHub.
Get-InstalledModule | Update-Module
All that's left is to wait while the magic happens...
V. Conclusion
This concludes the chapter on PowerShell modules. You can not only develop your own PowerShell modules, but also contribute to existing projects. If you want to get started, you should take a look at the Crescendo PowerShell module.
Today, there is a plethora of PowerShell modules to meet a wide variety of needs. PowerShell can interact with Excel spreadsheets, Word documents, 7-Zip archives, MySQL databases and Hyper-V virtual machines.