Creating your first PowerShell function
Table of Contents
I. Introduction
We're going to learn how to create our first PowerShell function, so that you can familiarize yourself with the concept. We'll look at the syntax of a function, using various examples.
II. Function syntax
In PowerShell, you can write functions without parameters and functions with parameters. It all depends on your needs. We'll look at both syntaxes.
A. Simple function without parameters
The basic syntax for defining a function is as follows:
function FunctionName {
# Instructions / code to execute when calling this function
}
For the function name, we'll try to follow Microsoft's good practice of using an approved verb (Get, New, Set, etc...) followed by a hyphen and then a noun.
For example, we could write the "Write-Hello" function, which has a single purpose: to return "Hello!" to the console each time it's called.
Here is the function code:
function Write-Hello {
Write-Output "Bonjour !"
}
In the script, to call this function, simply write :
Write-Hello
This means that if you write "Write-Hello" 3 times in our script, we'll call our function 3 times. Here's an example:
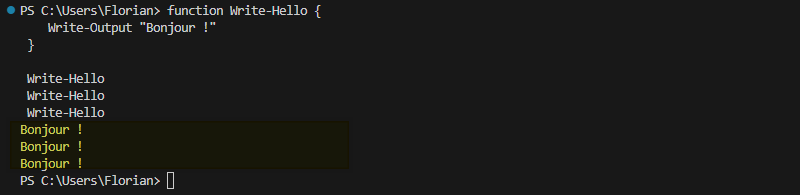
Note : in your code, the function must always be declared before it is called.
B. Function with parameters
The Write-Hello function we've just written is static, since it will always return the same result. We can't influence its behavior or the result returned, as it has no parameters. Like a PowerShell cmdlet, a PowerShell function can have one or more parameters.
In a function, a "param()" block must be included to declare parameters between parentheses.
function Write-Hello {
param($Parametre1,
$Parametre2
)
Write-Output "Bonjour !"
}
We'll see later that the parameter can have a default value, as well as a configuration (or "parameter settings"). This can be useful for making it mandatory.
Let's take our previous example a step further. We could add a "-Language" parameter to specify the two-letter language in which we'd like to say hello. Then, in the function, we'll need to parse the value associated with this parameter to return hello in the selected language. By default, we'll set the language to French.
Here is the code for this function, in which we use a switch to manage the different languages:
function Write-Hello {
param($Language = "FR")
Switch($Language)
{
"FR" { $Hello = "Bonjour" }
"EN" { $Hello = "Hello" }
"ES" { $Hello = "Hola" }
"IT" { $Hello = "Buongiorno" }
default { $Hello = "Bonjour" }
}
return $Hello
}
Now we can call our function, and depending on the language specified, a different value will be returned. If we don't specify a value, "FR" will be used by default, since we've specified "= "FR"" after the parameter name. If we specify a value not supported by the function, e.g. "CN", we'll still get a result which will be the one specified in the Switch "default" instruction (in other cases, this could generate a function execution error).
We need to specify "-Language" followed by a value, as this parameter is explicitly named "Language" when declared in the function.
Write-Hello
Write-Hello -Language "ES"
Write-Hello -Language "EN"
Here's the result, in pictures:

You should know that the "return" statement is used to indicate what the function should return. Here, it's to return the value of the "$Hello" variable, but it could be an array, an object, etc. Please note that the scope of the "$Language" variable is limited, since it exists only within the function.
C. Type a function parameter
Finally, we'll look at how to "type" a parameter in a function. This means that we're going to impose the type of a variable, without leaving it up to PowerShell.
Whether it's a function parameter or a variable in a script, the syntax is the same: the type must be specified between square brackets, before the variable name. If we want to use the "String" type for the "-Language" parameter, since we're expecting a string, we'll use this:
function Write-Hello {
param([string]$Language = "FR")
Switch($Language)
{
"FR" { $Hello = "Bonjour" }
"EN" { $Hello = "Hello" }
"ES" { $Hello = "Hola" }
"IT" { $Hello = "Buongiorno" }
default { $Hello = "Bonjour" }
}
return $Hello
}
III. Conclusion
In the next chapter, we'll take a closer look at the subject of PowerShell functions, in particular by adding checks on PowerShell function parameters.