Conditional structures: Switch
Table of Contents
I. Introduction
In this chapter, we'll learn how to use conditional structures based on the Switch statement, in PowerShell! The Switch is very useful when you need to execute a different action or assign a different value to a variable depending on an input value, and there are a multitude of possible values. In this particular case, the use of Switch is much more efficient and appropriate than a sequence of conditions in an "if-elseif-else" block: this will lighten the code and improve its readability.
In concrete terms, a Switch instruction will take an expression as input and compare the value of this expression with a list of cases (declared by you). If there is a full or partial match (depending on the switch configuration), the associated block of code will be executed. If no match is found, a default code block can be executed.
II. Getting started with Switch
To understand the benefits of the Switch instruction block, let's start by comparing it to the "if-elseif-else" conditions. It's not uncommon to want to execute a different action depending on the value of a variable. When you use a variable within a loop (in a "ForEach", for example), chances are that the value of the variable will be different from one loop turn to the next.
In this case, we can use if-elseif-else to execute different actions depending on the value being tested.
Which gives:
# Structure générale if-elseif-else
If("<condition 1>")
{
# bloc de code (instructions)
}
ElseIf("<condition 2>")
{
# bloc de code (instructions)
}
ElseIf("<condition 3>")
{
# bloc de code (instructions)
}
ElseIf("<condition 4>")
{
# bloc de code (instructions)
}
Else
{
# bloc de code (instructions)
}
Where the condition could be "my value = 1", "my value = 2", etc. When we want to act according to the value of a variable, we can use a switch. In this way, the "if-elseif-else" block mentioned above would become :
Switch ("<valeur à tester>")
{
"<condition 1>" { "bloc de code (instructions)" }
"<condition 2>" { "bloc de code (instructions)" }
"<condition 3>" { "bloc de code (instructions)" }
"<condition 4>" { "bloc de code (instructions)" }
Default { "bloc de code (instructions)" }
}
Where the value to be tested could be "1", "2", etc., and the condition simply each value. Formally, we can describe the syntax like this:
switch -paramètre (<valeur>) {
"Chaîne" | Nombre | Variable | { Expression } { Bloc de code à exécuter }
default { Bloc de code à exécuter }
}
To make it more evocative, let's take a concrete example.
We're going to define the variable $SoftwareName, which will contain the name of a software program that we'll enter in the PowerShell console (value retrieved with Read-Host). Next, we'll test this value using a switch. For example, if the value is "notepad", we'll run the notepad.exe application on the machine, while if the value is "powershell", we'll open a PowerShell console on the console, and so on... Clearly, a different action is executed depending on the input value. Then, as a default action (if we can't find the requested software, for example), we'll simply indicate"Sorry, I couldn't find this software".
Which gives:
# Exemple n°1
$NomLogiciel = Read-Host -Prompt "Quel logiciel souhaitez-vous lancer ?"
switch ($NomLogiciel)
{
"notepad" { Start-Process notepad.exe }
"powershell" { Start-Process powershell.exe }
"calc" { Start-Process calc.exe }
"regedit" { Start-Process regedit.exe }
Default { "Désolé, je n'ai pas trouvé ce logiciel" }
}
So, if we execute this piece of code and specify "notepad", the application will run on the local machine:
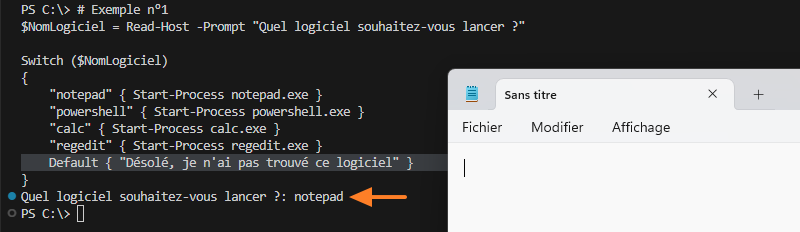
On the other hand, if we specify an unmanaged value, the instruction in"Default" will be executed:
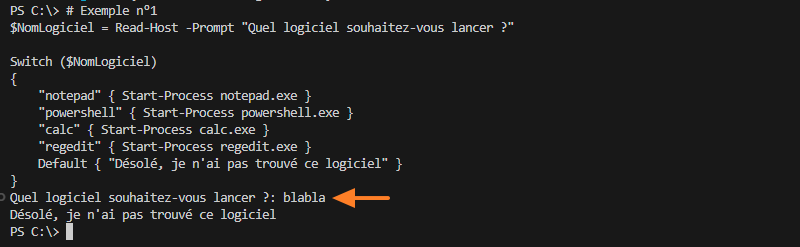
As it stands, it should be noted thatthere must be an exact match between the value to be tested and the values declared in the Switch for this to work. In other words, if we specify "notepa", Switch will not match "notepad", and so the associated block of code will not be executed. However, Switch is not case-sensitive by default.
III. Switch: testing two values
In this first example, we saw that it was possible to test one value. In a switch, you can test several values: not at the same time, but in turn. For example, we could adapt the previous example to ask for the name of a second piece of software.
Which gives:
# Exemple n°2A
$NomLogiciel1 = Read-Host -Prompt "Quel logiciel souhaitez-vous lancer ?"
$NomLogiciel2 = Read-Host -Prompt "Quel logiciel souhaitez-vous lancer ?"
switch ($NomLogiciel1,$NomLogiciel2)
{
"notepad" { Start-Process notepad.exe }
"powershell" { Start-Process powershell.exe }
"calc" { Start-Process calc.exe }
"regedit" { Start-Process regedit.exe }
"powershell_ise" { Start-Process powershell_ise.exe }
Default { "Désolé, je n'ai pas trouvé ce logiciel" }
}
On execution, we can see that PowerShell does indeed request two software names, and that the result will be different for each value.
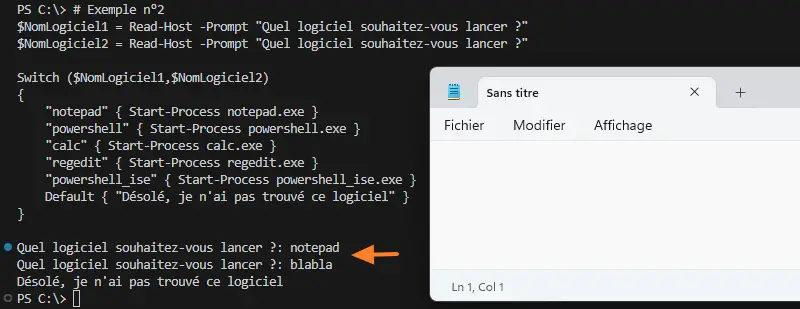
We have seen above that it is possible to indicate several input values. This also applies to the values to be compared in our switch block. For example, if we wish to open PowerShell ISE if the user specifies "powershell_ise" or "ise", we can write it this way to accept multiple values for the same block of code:
# Exemple n°2B
$NomLogiciel = Read-Host -Prompt "Quel logiciel souhaitez-vous lancer ?"
Switch ($NomLogiciel)
{
"notepad" { Start-Process notepad.exe }
"powershell" { Start-Process powershell.exe }
"calc" { Start-Process calc.exe }
"regedit" { Start-Process regedit.exe }
{"ise","powershell_ise"} { Start-Process powershell_ise.exe }
Default { "Désolé, je n'ai pas trouvé ce logiciel" }
}
IV. Switch parameters: wildcard, break, regex, etc.
So far, we've seen a very simple use of switch: define a value to test and compare this value with those defined in the switch. As I said earlier, this only works when there's a real match. To go a step further, switch supports several parameters:
- -Wildcard: allows the use of wildcard characters such as "*" and "?" in switch values, to enable partial matching. As a reminder, "*" is used to replace all characters, and there can be more than one, while "?" is used to replace a single character.
- -Regex: allows the use of regular expressions in switch values. Useful for creating complex correspondence models (see this tutorial on RegEx).
- -CaseSensitive: enables case sensitivity for value comparison
- -File: retrieves values from a file for comparison
In the example below, the"-wildcard" parameter is used to declare the values"N?TEPAD" and"note*" instead of"notepad", which was used previously. The syntax of the switch is very slightly different, but this will influence its operation.
# Exemple n°3
$NomLogiciel = "notepad"
switch -Wildcard ($NomLogiciel)
{
"N?TEPAD" { Start-Process notepad.exe }
"note*" { Start-Process notepad.exe }
"calc" { Start-Process calc.exe }
"regedit" { Start-Process regedit.exe }
Default { "Désolé, je n'ai pas trouvé ce logiciel" }
}
If the software name is "notepad", what happens?
The software will be opened twice! Yes, twice!
In fact, there's a first match between "notepad" and "N?TEPAD", so the associated block of code will be executed: notepad.exe will be launched on the machine. Then, there's a second match between "notepad" and "note*", so notepad.exe will be launched a second time.
Interesting, because it means that all values are tested: when a match is found, we continue testing, we don't exit the switch! Unlike an "if-elseif-else" conditional block! That's a notable difference.
However, we can adjust the syntax of our switch block to exit when we find a match with a value. In this case, we add a "break" in the code block for each value.
Which gives:
# Exemple n°4
$NomLogiciel = "notepad"
switch -Wildcard ($NomLogiciel)
{
"NOTEPAD" { Start-Process notepad.exe ; break }
"note*" { Start-Process notepad.exe ; break }
"calc" { Start-Process calc.exe ; break }
"regedit" { Start-Process regedit.exe ; break }
Default { "Désolé, je n'ai pas trouvé ce logiciel" }
}
With this new syntax, and still using the "notepad" value, the notepad.exe application will be executed just once!
Note: if you want to use a regular expression, simply indicate the pattern as a value in the switch list (just like "NOTEPAD", "note*", etc.).
V. Switch - Complete example
Finally, here's a more complete example to show you that we can execute more complex blocks of code in a switch: there's no real restriction, as there is in an"if-elseif-else" conditional block.
In the example below, the variable $Software contains a list of software programs, and the switch contains installation instructions for each program. So, if $Software equals "Firefox", then the script will install Firefox on the machine (by retrieving the local source).
# Exemple n°5
$Logiciels = @("Firefox","7-Zip")
switch($Logiciels)
{
"Firefox" {
$Installeur = "C:PSFirefox.msi"
If(Test-Path $Installeur)
{
Write-Host "L'installation de Firefox ESR va débuter" -ForegroundColor Green
Start-Process $Installeur -ArgumentList "/quiet" -Wait
}
Else
{
Write-Host "Package MSI de Firefox introuvable ($Installeur)" -ForegroundColor Red
}
}
"7-ZIP" {
$Installeur = "C:PS7z-x64.msi"
If(Test-Path $Installeur)
{
Write-Host "L'installation de 7-Zip va débuter" -ForegroundColor Green
Start-Process $Installeur -ArgumentList "/quiet" -Wait
}
Else
{
Write-Host "Package MSI de 7-Zip introuvable ($Installeur)" -ForegroundColor Red
}
}
Default { "Désolé, je n'ai pas trouvé ce logiciel" }
}
VI. Conclusion
After reading this chapter, you'll be able to put the "switch" into practice in your PowerShell scripts! The "switch" is capable of meeting a wide range of needs, from the simple (comparison of values) to the complex (use of wildcards, regexes), by declaring a set of values. In addition to your values, remember to declare and add code in the "Default" case to handle all other cases.
Now we're going to start learning about loops in PowerShell.