Compare objects with Compare-Object
Table of Contents
I. Introduction
The "Compare-Object" PowerShell command is very useful for comparing two objects, such as two variables, the contents of two files or the contents of two folders. On several occasions, I've used this command to compare two CSV files.
When we compare two objects with "Compare-Object", the command returns :
- Identical elements between the two files
- Elements present in the reference object but absent from the second object
- Elements not present in the reference object, but present in the second object
In this chapter, you'll learn how to compare two objects in PowerShell with "Compare-Object".
II. Compare-Object: compare two strings
The "Compare-Object" cmdlet can, quite simply, compare two strings. This will help us to understand the syntax of this command.
To compare two strings, simply specify them after "Compare-Object" :
Compare-Object "www.it-connect.fr" "IT-Connect"
If the strings are equal, the command will return nothing. If you want to include equal elements, add the "-IncludeEqual" parameter.
Compare-Object "www.it-connect.fr" "WWW.IT-CONNECT.COM" -IncludeEqual
As we can see from this example, the comparison is not case-sensitive. If it's important, we can add the "-CaseSensitive" parameter to take case into account.
Compare-Object "www.it-connect.fr" "WWW.IT-CONNECT.COM" -IncludeEqual -CaseSensitive
Which gives:
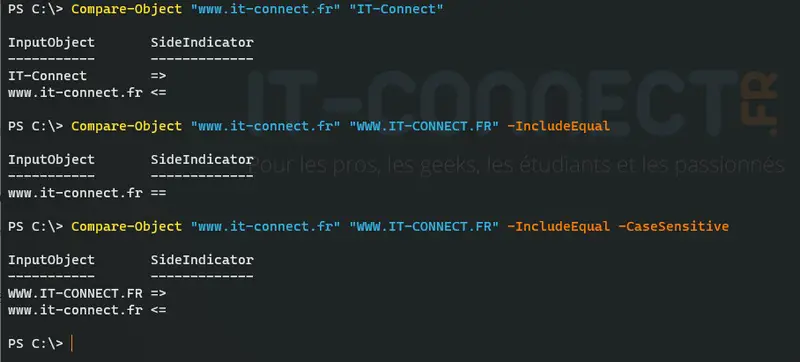
Following this introduction, let's move on to a much more useful example: comparing CSV files with PowerShell and "Compare-Object".
III. Compare-Object: comparing two CSVs
For this example, we will use the following two CSV files:
- CSV1.csv
Countries; France; Costa Rica; Canada; Belgium; Switzerland;
- CSV2.csv
Countries; France; Costa Rica; Spain; Belgium; Iceland; Colombia;
Each CSV file contains a single column named "Country", but we could have several columns. We'll compare these two files to easily identify the differences.
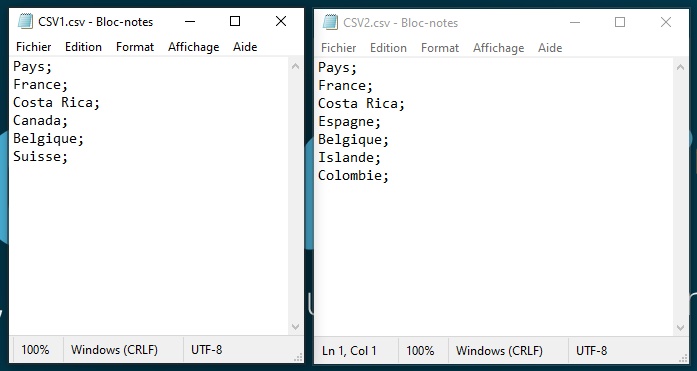
We'll start by importing the contents of our CSV files into the "$CSV1" and "$CSV2" variables. To do this, we'll use the "Import-CSV" cmdlet, which performs this function.
$CSV1 = Import-CSV "C:TEMPCSV1.csv" -Delimit ";" $CSV2 = Import-CSV "C:TEMPCSV2.csv" -Delimit ";"
Next, we'll compare the "$CSV1" and "$CSV2" variables. The CSV1 file will be our reference object, which we'll associate with the "-ReferenceObject" parameter, while the "difference" object will be the CSV2 file. We also need to specify the property on which to base the comparison, in this case our "Country" column.
This gives the following command:
Compare-Object -ReferenceObject $CSV1 -DifferenceObject $CSV2 -Property Country
The following result is obtained:
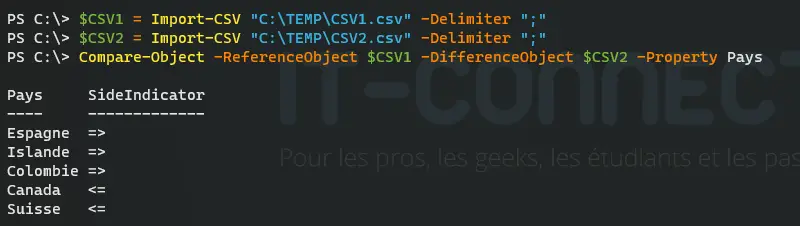
The "SideIndicator" property lets you know which side the :
- "=>": refers to a value present in the "-DifferenceObject" (CSV2), but absent in the "-ReferenceObject" (CSV1).
- "<=": refers to a value present in the "-ReferenceObject" (CSV1), but absent in the "-DifferenceObject" (CSV2).
If we want to know only the values present in the CSV1 file, but absent from the CSV2 file, we can add a filter based on the value of the"SideIndicator" property using"Where-Object".
Compare-Object -ReferenceObject $CSV1 -DifferenceObject $CSV2 -Property Pays | Where-Object { $_.SideIndicator -eq "<=" }
To reverse, simply change the direction of the arrow in the filter.

We can also display only common values between the two files by adding the"-ExcludeDifferent" parameter to exclude different values:
Compare-Object -ReferenceObject $CSV1 -DifferenceObject $CSV2 -Property Country -ExcludeDifferent

Please note that it is possible to compare several columns, and not just one, as in this case with the "Country" column. This means that a row can appear several times (in both directions) if the value of a column changes in one file, but not in the other.
Here's an example with the addition of the "Continent" column to our CSV files. In this example, for the country "Colombia", the continent value evolves between the two files, so the line appears once in the "=>" direction and a second time in the other direction "<=".
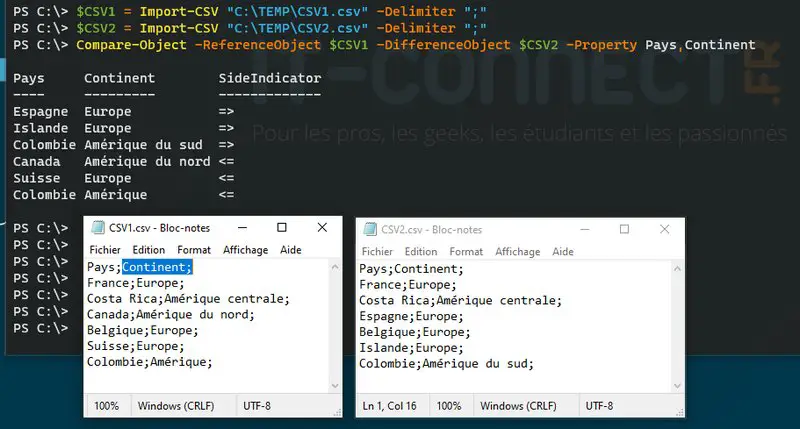
IV. Compare-Object: comparing the contents of two folders
To conclude this introduction to Compare-Object, let's take another concrete example: comparing the contents of two folders. The aim: to identify files present in one folder, but not in the other.
Here are the two folders used for this example:
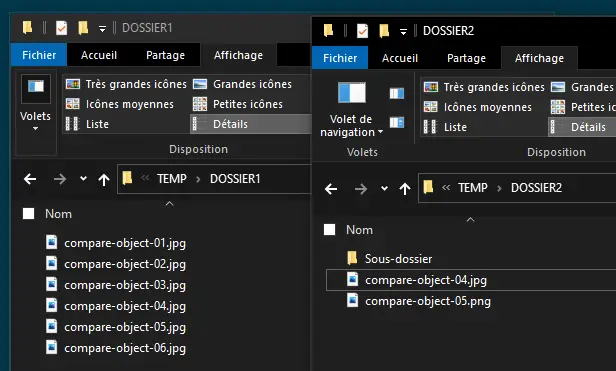
Let's start by recursively retrieving the list of items with the"Get-ChildItem" command.
$DOSSIER1 = Get-ChildItem "C:TEMPDOSSIER1" -Recurse $DOSSIER2 = Get-ChildItem "C:TEMPDOSSIER2" -Recurse
Next, we use the "Compare-Object"command on the same principle as above, without specifying a property.
Compare-Object -ReferenceObject $DOSSIER1 -DifferenceObject $DOSSIER2
We directly obtain the full paths to files that are missing in one folder or another.
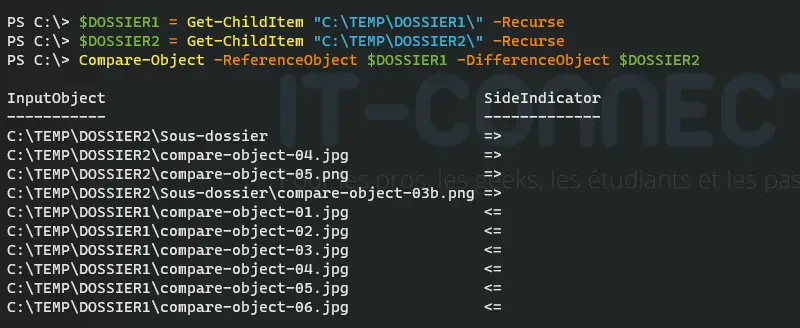
We can also use the "-Property" parameter of "Compare-Object" to compare the last modification dates of the files. This requires reading the "LastWriteTime" attribute. We'll also add the file name (not the full name) so that the output doesn't contain only the value of "LastWriteTime".
Compare-Object -ReferenceObject $DOSSIER1 -DifferenceObject $DOSSIER2 -Property Name,LastWriteTime -IncludeEqual
In this way, we can identify the most recent files more easily thanks to this differential.
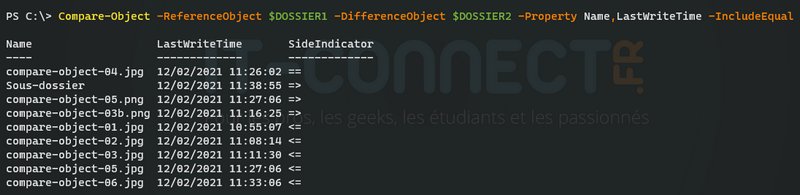
Now it's your turn! Feel free to try out these examples and practice on your own folders and files.