Automatic variables
Table of Contents
I. Introduction
Having studied the notion of variable and learned how to create our own variables, let's move on to automatic variables. In PowerShell, in addition to the variables you create yourself, there are automatic variables, created and managed by PowerShell itself. These variables provide useful information on the current state of your PowerShell session and are invaluable when writing a PowerShell script. You can view these variables, but you cannot modify them.
In this chapter, we'll explore some of these automatic variables and see how they can be used.
II. The $_ variable
The "$_" variable is probably the best-known and most useful of the automatic variables. It represents the current object in the pipeline. When we use a loop, such as "ForEach-Object", we use this variable. This is also the case when creating a filter with "Where-Object". Each time,"$_" represents the current object on which the loop is working.
Here's an example of how to obtain a list of all local users activated on the local machine:
Get-LocalUser | Where-Object { $_.Enabled -eq "True" }
Specifying "$_.Enabled" applies the filter to the "Enabled" property of each object, i.e. each user to be processed. As you may have guessed, using "$_" selects the entire object, while "$_.<property name>" selects only one property.
II. The $PSVersionTable and $PSHOME variables
The "$PSVersionTable" variable contains information about the PowerShell version you're using, including major, minor and revision. In addition, the "$PSHOME" variable contains the path to the directory where PowerShell is installed.
You can try these two variables:
# Displays the PowerShell version
Write-Host $PSVersionTable.PSVersion
# Displays the PowerShell installation path
Write-Host $PSHOME
Which gives:

III. The $Error variable
The"$Error" variable contains a list of the latest errors that have occurred in the PowerShell session. It is very useful for obtaining the list of errors following the execution of a script, as well as for error management. This automatic variable corresponds to an array (the first value of an array is associated with index 0). The first error, i.e. the most recent, is accessible via"$Error[0]", the second via"$Error[1]", and so on.
When we open a new PowerShell console, this variable is empty. As soon as an error occurs, it is added to the "$Error" array. The example below shows this explicitly:
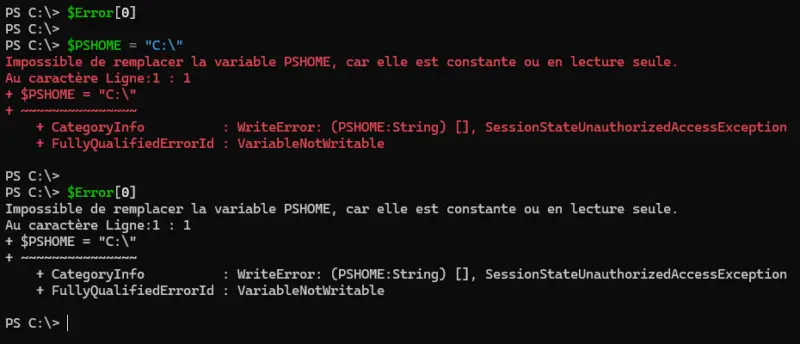
IV. The variable $?
The"$?" variable, not to be confused with "$_", is another essential automatic variable in PowerShell. It contains the status of the last operation (command executed). If the last command was executed successfully, "$?" will equal "True", otherwise, if it failed, "$?" will equal "False". This automatic variable is of Boolean type.
This is particularly useful for checking whether an operation has been carried out as planned, and for handling errors. This allows an action to be executed only if the previous action was successful.
# Recover the state of a non-existent process (to generate a failure)
Get-Process -Name "ProcessusInexistant"
# Checks if the command was successful
if ($?) {
Write-Host "La commande a été exécutée avec succès "
} else {
Write-Host "La commande a échouée"
}
In this example, the "Get-Process " command will attempt to find a process that doesn't exist, which will fail. Consequently, the value of"$?" will be "False" and the sentence"Command failed" will be displayed.
V. The $true, $false and $null variables
These variables represent the Boolean values true ($true) and false ($false), as well as the null value ($null). They are often used in conditional structures, which we'll look at later (if, else).
Here's an example:
if ($MaVariable -eq $null) {
Write-Host "The variable is null"
}
In the example above, the"$MyVariable" variable contains no value, so it's considered null.
VI. The $PSScriptRoot variable
The"$PSScriptRoot" variable is an automatic variable containing the directory path from which the current script is executed. This variable is very useful when you need to refer to other files or scripts located in the same directory as the main script. Indeed, you may not know in advance the directory in which the script will be stored (especially for scripts you're going to share), so the "$PSScriptRoot" variable will tell you.
Suppose, for example, you have a PowerShell script that needs to read a text file located in the same directory as the script file itself. You can use the "$PSScriptRoot" variable to construct the path to the :
# Build path to file
$FichierChemin = $PSScriptRoot + "" + "MonFichier.txt"
# Read file contents
$FichierContenu = Get-Content -Path $FichierChemin
This implies that this piece of code must be executed via a PS1 script, otherwise the "$PSScriptRoot" variable will be empty, as it will be impossible to point to a script file. If the script is stored in the "C:PS" directory, then the "$PSScriptRoot" variable will be equal to "C:PS". If the script is moved, then the path will adapt automatically.
In addition, the "$PSCommandPath" variable contains the full path to the directory and the script name. If the "Demo.ps1" script is stored in "C:PS", then this variable will have the value "C:\PS\Demo.ps1".
VII. The $IsWindows, $IsLinux and $IsMacOS variables
Since PowerShell 7.0, the PowerShell language supports three additional automatic variables: "$IsWindows", "$IsLinux" and "$IsMacOS". These are very useful for determining the operating system (OS) on which PowerShell is run. As their names suggest, these variables will return "True" if PowerShell is running on the corresponding OS, and "False" otherwise.
In other words, if PowerShell is run on Windows, the $IsWindows variable will be set to "True", while the other two variables will be set to "False". The mechanics are the same with "$IsLinux" for Linux and "$IsMacOS" for MacOS. For example, this can enable you to have a single script that adapts from one system to another: the access paths being different between these OSes.
VIII. Conclusion
As we've just seen, each automatic variable plays a very different role. PowerShell's automatic variables play a valuable role, providing a convenient means of accessing a variety of information. By using them judiciously, you can make your scripts more flexible, especially when it comes to adapting to the execution environment. There are many others, including the following variables :
- $$: contains the value returned by the last PowerShell command executed
- $Home : contains the path to the current user's profile directory
- $Host : contains some information about the host system
- $PSCulture : contains the language used by the user (fr-FR, on a French system)
- $PID: contains the process number of the current PowerShell session
- $PROFILE: contains the path to the current user's PowerShell profile file