Arithmetic operators
Table of Contents
I. Introduction
This chapter looks at the arithmetic operators that can be used with PowerShell, enabling us to perform mathematical operations such as addition, subtraction, division and multiplication.
Although these operators are designed to perform operations on numbers, we'll see that addition is particularly interesting with strings to perform what's known as string concatenation.
II. The list of arithmetic operators
Below is a list of the 5 arithmetic operators you can use with PowerShell :
Operator | Description |
---|---|
+ | Addition Concatenate strings Add numbers |
- | Subtraction Subtracting numbers |
* | Multiplication Multiplying numbers |
/ | Division Dividing numbers |
% | Modulo Remainder of a division |
To remember which characters to use for each operation, simply remember how to write these calculation operations in the Windows Calculator, or in Excel, for example.
We'll now look at how to use these operators.
III. Addition and concatenation
A. Addition
For this first example, I'd like to draw your attention to the fact that the variable type is very important when it comes to manipulating numbers. Indeed, to add, subtract, etc... values from each other, they must be of type "[int] " for "integer", i.e. an integer number, or of type "[Double]" for a decimal number.
We'll define two variables $Digit1 of type "[string]"(i.e. a character string) and $Digit2 of type "[int]".
[string]$Digit1 = 1 [int]$Digit2 = 2
When we need to add two values, we simply add a "+" between the two values, or the two variable names. So, if we add the variables $Figure1 and $Figure2, we should get "3". Let's give it a try.
$Digit1 + $Digit2
PowerShell returns the following result:
12
It's not the result we expected. But it makes sense. We don't get "3" but "12", which simply corresponds to the values of my two variables written in succession. In other words, PowerShell didn't do the math! In fact, it's because our variable $Chiffre1 has the type "[string]"! If we perform the same exercise with the "[int]" type on both variables, we'll see that it works:
[int]$Digit1 = 1 [int]$Digit2 = 2 $Digit1 + $Digit2
This time, the result is correct:
3
Here's a pictorial summary of what we've just done:

Note that I've forced the "[int]" type on my two variables, but this isn't mandatory. PowerShell is clever enough to type variables itself according to the data assigned (generally, this works well). Let's take an example:
number3 = 3
If we look at the type of this variable, we'll see that it's "Int32", which corresponds to the type of the integer "category".
$figure3.GetType()

Remember this: to add values in PowerShell, you need to add the "+" character between values, whether two or more.
B. String concatenation
Concatenating strings with the "+" operator will act on strings in text format, as if we were trying to add them together, or merge them if you prefer. We're going to define two variables: one with the first name and another with the last name. Which gives:
# Prénom
$prenom = "Florian"
# Nom
$nom = "Burnel"
Now, if we want to concatenate these two values to make a single value that will be stored in a new variable named "$lastname", we'll use this syntax:
$prenomnom = $prenom + $nom
The problem is that this syntax will return the following result:
FlorianBurnel
In other words, there's a space missing between the two values! To improve the result, we're going to concatenate these two values with a space that will be positioned between the two strings. This gives us the following syntax:
$prenomnom = $prenom + " " + $nom
Since there's a space between the quotation marks, we get a cleaner output:
Florian Burnel
Now, if we just want to write the first and last name in the console, we can use this syntax without the "+" :
Write-Host $prenom $nom
We could imagine the concatenation between a string and our two variables like this:
Write-Host "Je m'appelle $prenom $nom !"
Which gives:
Je m'appelle Florian Burnel !
There are other methods for concatenating strings, such as the "-join" and "-f" operators. To find out more, see this article:
IV. Subtraction
To subtract two values, it's the same principle: the variable type must allow it, and then we'll use the "-" sign instead of "+", as we learned at school. Just try :
2 - 2
You'll get "0". On the same principle as before, we can use two variables.
$digit1 = 10 $digit2 = 1 $digit1 - $digit2
If the result of the subtraction is a negative value, less than zero, this is not a problem. PowerShell will return this negative value.
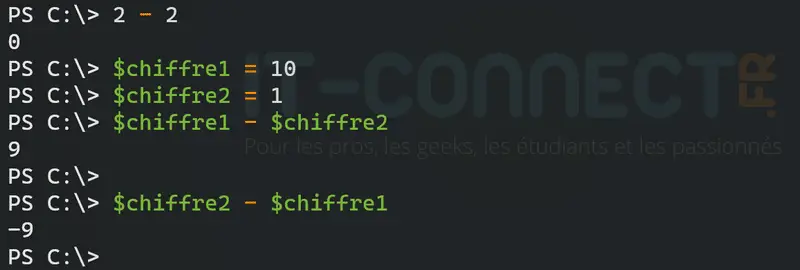
V. Division and modulo
PowerShell not only divides one value by another, but also returns the modulo, i.e. the remainder of a division. The "/" character is used to separate the values that make up a division, while the "%" character is used for modulo.
Here are a few examples:
# Example 1 - Division 10 / 2 = 5 # Example 2 - Division $digit1 = 10 $digit2 = 3 $digit1 / $digit2 = 3,33333333333333 # Example 3 - Division (10 + 10) / 2 = 10 # Example 4 - Modulo 10% 3 = 1 # Example 5 - Modulo (12 / 2) % 2 = 0
VI. Multiplication
We'll finish with multiplication, using the "*" character. Here's a series of examples:
# Example n°1 10 * 2 = 20 # Example n°2 $digit1 = 10 $digit2 = 3 $digit1 * $digit2 = 30
This last example shows that we can combine the use of different operators in the same mathematical operation.
((10 * 10) - 10 + 100) / 2
= 95
Parentheses are important too, just as they are when we do math for real: the same rules apply. Take a look at these two examples to understand the importance of parentheses:

VII. Going further with [System .Math]
Finally, and to take things a step further, the .NET framework (and .NET Core) includes a class named [System .Math], which contains 45 methods for using various calculation functions.
We can list all the methods in this class with the following command:
[System .Math] | Get-Member -Static -MemberType Methods
As you can see, the list is quite extensive:
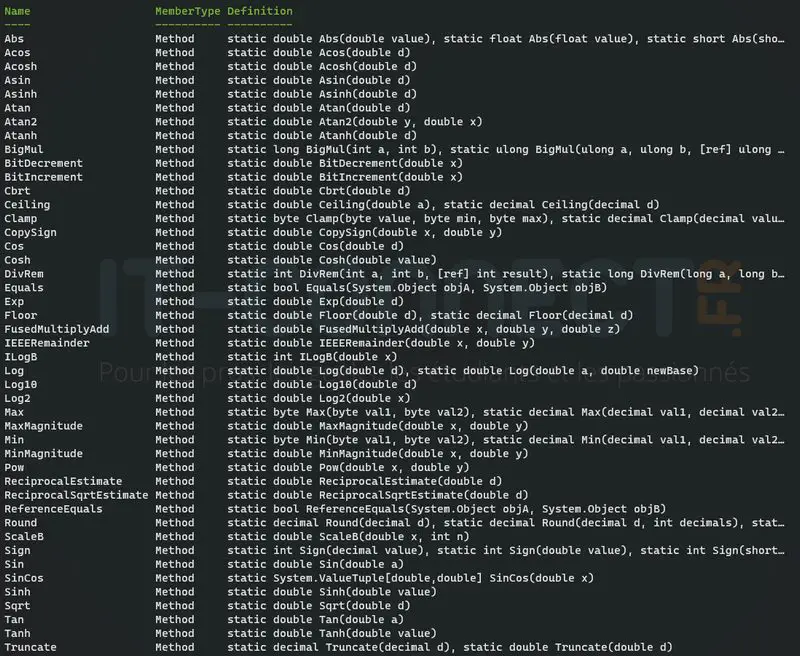
For example, the"Pow()" method calculates the power, e.g."2 to the 8th power", so you need to specify both numbers in the method. Here's an example:
[math]::Pow(2,8)
# Result = 256

If you wish to compare two values to obtain the maximum value between them, you can use the"Max()" method by specifying the two values (directly or via a variable):
$figure1 = 100
$figure2 = 95
[math]::Max($figure1,$figure2)
# Result = 100
In the same spirit, you can replace "Max" with "Min" to obtain the lower of the two values.
To round a value up or down to the nearest decimal place, use the Round() method. We specify a number as a parameter and the method will round it up or down, depending on what's most relevant. Here's the syntax:
[math]::Round(10.7)
# Result : 11
We can see that with the value "10.3", it's rounded to 10 and not 11, which is logical. For the value "10.5", we get "10", while for "10.51" we get the value "11".

We've just seen the essentials when it comes to performing mathematical operations with PowerShell. If you want to go further, take a look at the various methods in the .NET "System.Math" class.
VIII. Conclusion
PowerShell is very comfortable performing mathematical operations, from simple addition and concatenation to more advanced operations.