Analyzing an object with Get-Member
Table of Contents
I. Introduction
Having seen how to request help from PowerShell with the "Get-Help" cmdlet or by consulting the online help on the Microsoft Learn site, we'll now look at how to explore a cmdlet with "Get-Member" to better understand how it works.
The "Get-Member" cmdlet allows us to retrieve information about the object returned by a PowerShell command, including a list of all available properties, methods and events.
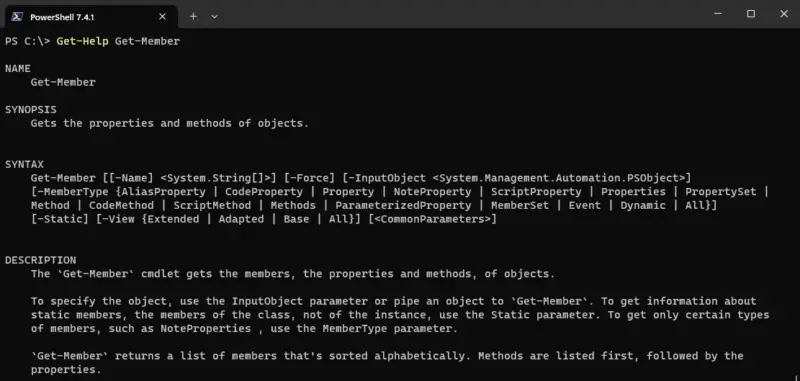
II. Properties and methods
When working with PowerShell, two key concepts we use regularly are properties and methods. It's important to understand both concepts. As PowerShell is an object-oriented language, each object has properties and methods that enable us to interact with it.
A. Properties
Properties are the characteristics of an object, and they can be more or less numerous from one object to another, depending on the amount of information to be stored. To fully understand the principle of properties, let's illustrate them with an example.
If we have a "Car" object, it could have a set of properties to describe the car: "Make", "Model", "Color", "Power", "Engine", etc. To access an object's properties in PowerShell, we use pointed notation. For example, if "Car" is our object, we can access the car's make using this type of notation: "Car.Color".
If we take the example of an object representing a Windows service, it will have several properties: a technical name, a display name, a state, a startup type, etc... These are the properties.
Run the following command (to obtain a list of services registered on the local machine):
Get-Service
The objects returned contain several properties, including three that are visible in the console: Status, Name and DisplayName. Later, "Get-Member" will give us a list of all available properties.
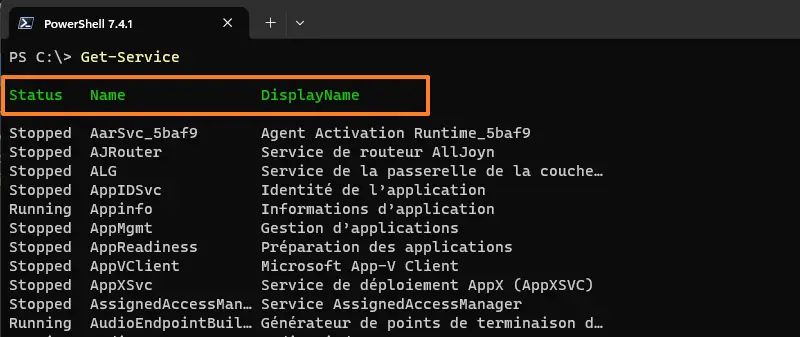
If we wish to retrieve only the value of a particular property, we can use dotted notation. Since we're using the cmdlet name directly, it should be enclosed in brackets. Here are several examples with different property names:
# Récupérer uniquement la valeur de la propriété DisplayName
(Get-Service).DisplayName
# Récupérer uniquement la valeur de la propriété Name
(Get-Service).Name
# Récupérer uniquement la valeur de la propriété Status
(Get-Service).Status
Here is an example of a return:
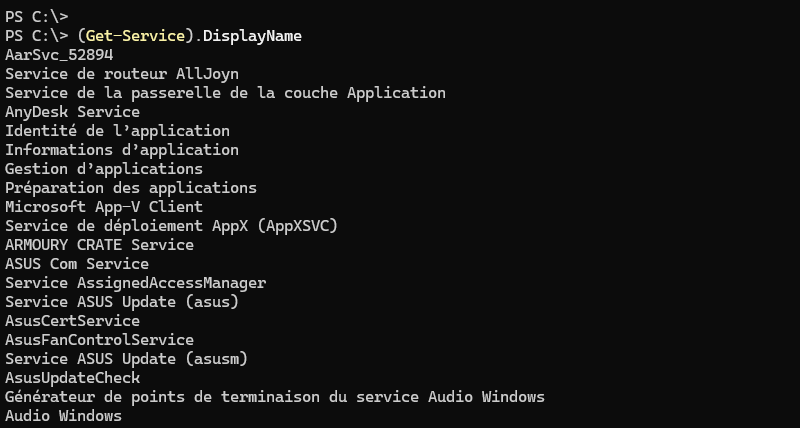
B. Methods
Methods are actions that can be performed on an object. In other words, it's a way of interacting with an object to change its state or perform a specific task. Taking our example of the "Car" object, we can think of several methods: "Drive", "Brake", "Honk", etc. To call and execute a method with PowerShell, we can also use dotted notation, followed by parentheses. For example: "Car.Drive()".
It's important to note that not all methods change the state of the object. Some methods may simply return information about the object without modifying it, or perform manipulation on a string.
If we return to the previous example based on "Get-Service", this cmdlet supports several methods, including "Start" and "Stop", which allow you to start and stop a service respectively. Thus, we can start the Windows Update service (associated with the name "wuauserv") with this command:
(Get-Service -Name "wuauserv").Start()
There are other popular and very practical methods for manipulating strings. For example, to change a string to uppercase(ToUpper()), to lowercase(ToLower()), to extract a part of it(Substring()), or to split it into several parts(Split()). When we're manipulating first and last names in order to "construct" identifiers corresponding to a specific format, these methods come in very handy!
Here are some examples in the image below:
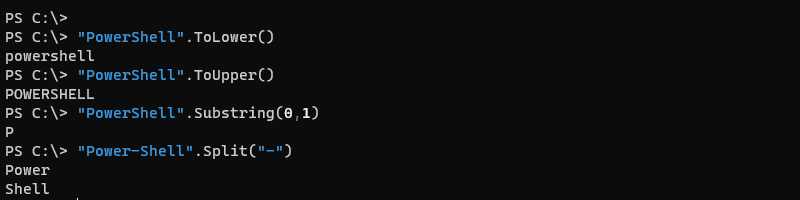
To learn more about this subject, read these articles:
- PowerShell and the Split() method
- PowerShell and the Substring() method
- PowerShell and the Trim() method
III. Using Get-Member
How do I get a list of available properties and methods? This is the question that the "Get-Member" cmdlet (alias "gm") will answer.
First of all, "Get-Member" is always used this way:
<Commande> | Get-Member
This is understandable, as we need to tell it an object to analyze. It will retrieve it via the "|" character, which we call pipeline and which will be explained in detail in the next chapter.
To obtain the list of properties and methods available for the "Get-Service" command, we will execute the following:
Get-Service | Get-Member
Below is an extract of the returned result. We can see that the"MemberType" column returns a set of methods and properties (as well as property aliases). We can see the two previous methods:"Start()" and"Stop()". Note also that the list contains much more than 3 properties (those returned by default by the "Get-Service" command).
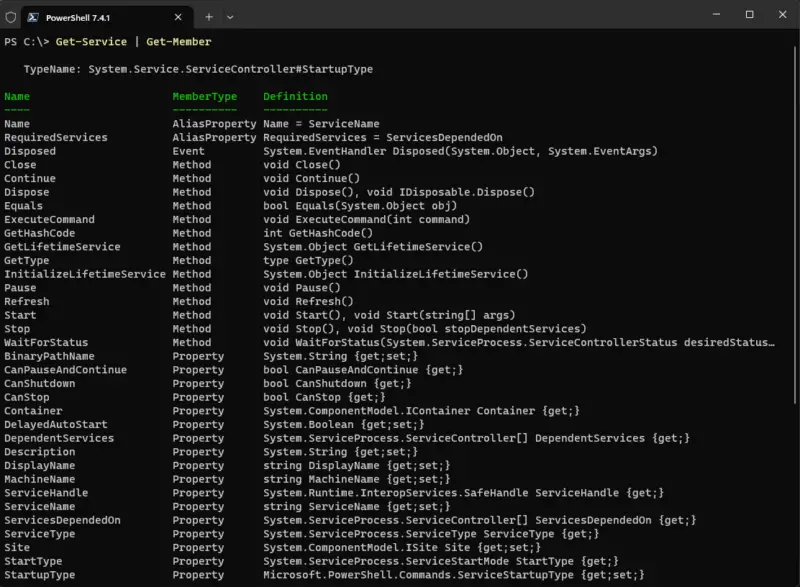
If we want to display the"DisplayName" and"StartType" properties to view the service's display name and startup type, we can do the following:
Get-Service | Format-Table DisplayName, StartType
The"Format-Table" command returns a table with the two properties shown:
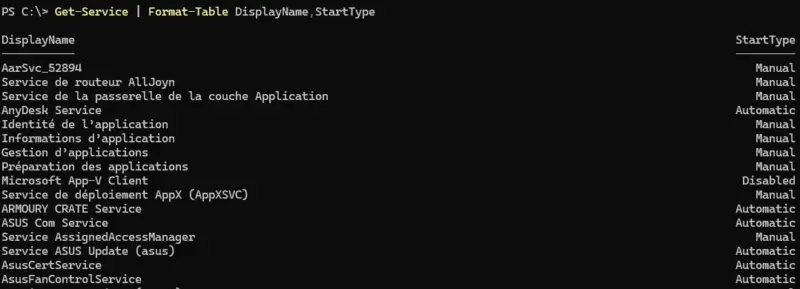
We can add the"MemberType" parameter to obtain only the list of methods or properties.
Get-Service | Get-Member -MemberType Method
Get-Service | Get-Member -MemberType Property
IV. Conclusion
Understanding properties and methods is essential to working effectively with PowerShell, as they represent the richness of PowerShell objects. As we've seen, the "Get-Member" cmdlet plays an invaluable role in displaying the properties and methods relating to an object.